本文为本人自学 Mybatis 的学习笔记
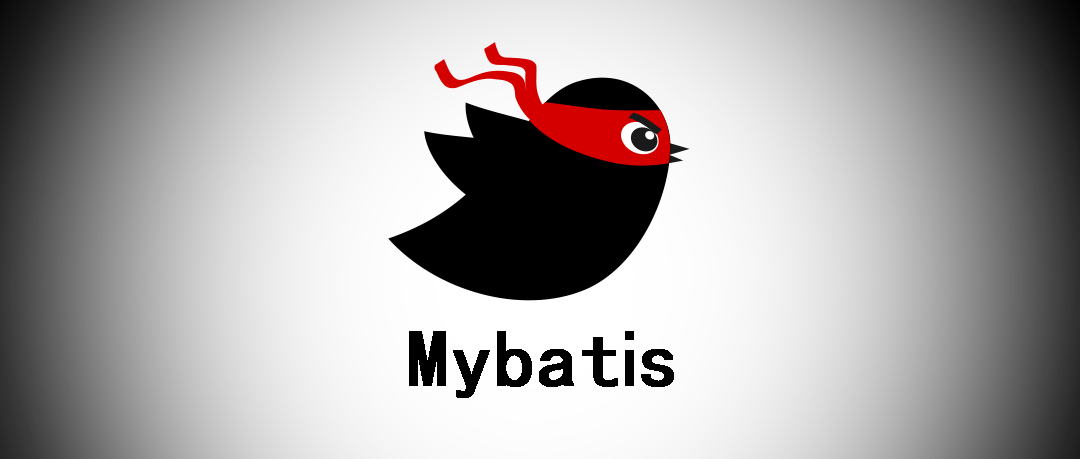
Mybatis
简介
为什么要用
特点
快速上手
搭建环境
搭建Mysql表
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17CREATE DATABASE `mybatis`;
USE `mybatis`;
CREATE TABLE `USER`
(
`id` INT ( 20 ) NOT NULL PRIMARY KEY,
`name` VARCHAR(30) DEFAULT (NULL),
`password` VARCHAR(30) DEFAULT (NULL)
) ENGINE = INNODB DEFAULT CHARSET = utf8;
INSERT INTO `user` (`id`, `name`, `password`)
VALUES (1, 'admin', '000000'),
(2, 'test1', '000000'),
(3, 'test2', '000000'),
(4, 'test3', '000000'),
(5, 'test4', '000000');新建maven项目
并在 maven 中引入如下依赖
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.11</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.7</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
</dependencies>配置maven资源路径
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20<build>
<resources>
<resource>
<directory>src/main/resources</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>false</filtering>
</resource>
</resources>
</build>
写第一个mybatis Demo
- 在 resources 中新建核心配置文件 mybatis-config.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
<configuration>
<environments default="development">
<environment id="development">
<transactionManager type="JDBC"/>
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/mybatis?useSS=true&useUnicode=true&characterEncodeing=UTF-8"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</dataSource>
</environment>
</environments>
</configuration> - 新建工具类,用于获得 SqlSessionFactory
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27package com.wx.learn.util;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import java.io.IOException;
import java.io.InputStream;
public class MybatisUtils {
private static SqlSessionFactory sqlSessionFactory;
static {
try {
String resource = "mybatis-config.xml";
InputStream inputStream = Resources.getResourceAsStream(resource);
sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream);
} catch (IOException e) {
e.printStackTrace();
}
}
public static SqlSession getSqlSession() {
return sqlSessionFactory.openSession();
}
} - 编写主体代码
实体类
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47package com.wx.learn.pojo;
public class User {
int id;
String name;
String password;
public User(int id, String name, String password) {
this.id = id;
this.name = name;
this.password = password;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String toString() {
return "User{" +
"id=" + id +
", name='" + name + '\'' +
", password='" + password + '\'' +
'}';
}
}接口
1
2
3
4
5
6
7
8
9package com.wx.learn.dao;
import com.wx.learn.pojo.User;
import java.util.List;
public interface UserDao {
public List<User> getUserList();
}mapper 文件
UserMapper.xml
1
2
3
4
5
6
7
8
9
10
<mapper namespace="com.wx.learn.dao.UserDao">
<select id="getUserList" resultType="com.wx.learn.pojo.User">
select * from mybatis.user
</select>
</mapper>编写测试类
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21package com.wx.learn.dao;
import com.wx.learn.pojo.User;
import com.wx.learn.util.MybatisUtils;
import junit.framework.TestCase;
import org.apache.ibatis.session.SqlSession;
import java.util.List;
public class UserDaoTest extends TestCase {
public void testUserDao() {
SqlSession sqlSession = MybatisUtils.getSqlSession();
UserDao userDao = sqlSession.getMapper(UserDao.class);
List<User> users = userDao.getUserList();
for (User u : users) {
System.out.println(u.toString());
}
sqlSession.close();
}
}在
核心配置文件 mybatis-config.xml
中注册UserMapper.xml
1
2
3<mappers>
<mapper resource="com/wx/learn/dao/UserMapper.xml"/>
</mappers>运行成功输出结果
1
2
3
4
5User{id=1, name='admin', password='000000'}
User{id=2, name='test1', password='000000'}
User{id=3, name='test2', password='000000'}
User{id=4, name='test3', password='000000'}
User{id=5, name='test4', password='000000'}
增删改查
- 在接口中增加 以下声明
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42package com.wx.learn.dao;
import com.wx.learn.pojo.User;
import java.util.List;
public interface UserMapper {
/**
* 查询所有 User
* @return
*/
public List<User> getUserList();
/**
* 根据Id查询User
* @param id
* @return
*/
public User getUserById(int id);
/**
* 向数据库 insert 传入的User
* @param user
* @return
*/
public int addUser(User user);
/**
* 更新传入的User
* @param user
* @return
*/
public int updateUser(User user);
/**
* 根据传入的Id删除User
* @param id
* @return
*/
public int deleteUser(int id);
}
查
- 根据Id查询User
1
2
3<select id="getUserById" parameterType="int" resultType="com.wx.learn.pojo.User">
select * from mybatis.user t where t.id = #{id}
</select>
-
1 |
|
增
- 向数据库 insert 传入的User
1
2
3<insert id="addUser" parameterType="com.wx.learn.pojo.User">
insert into mybatis.user(id, name, password) VALUE (#{id}, #{name}, #{password})
</insert>
-
1 |
|
改
- 更新传入的User
1
2
3<update id="updateUser" parameterType="com.wx.learn.pojo.User">
update mybatis.user t set t.name=#{name}, t.password=#{password} where t.id = #{id}
</update>
-
1 |
|
删
- 根据传入的Id删除User
1
2
3<delete id="deleteUser" parameterType="int" >
delete from mybatis.user where id = #{id}
</delete>
-
1 |
|
Spring 整合Mybatis
方式1
https://www.bilibili.com/video/BV1WE411d7Dv?p=24
方式2
https://www.bilibili.com/video/BV1WE411d7Dv?p=25
Spring-Mybatis中的事物管理
声明试
https://www.bilibili.com/video/BV1WE411d7Dv?p=27